What makes Node work?
Node.js
The bot is written in JavaScript using Node.js.
Node.js is a JavaScript runtime built on Chrome's V8 JavaScript engine. As an asynchronous event-driven JavaScript runtime, Node.js is designed to build scalable network applications.Node.js is also the reference behind bots likeness.
in this "hello world" example, many connections can be handled concurrently. Upon each connection, the callback is fired, but if there is no work to be done, Node.js will sleep.
const http = require('http');
const hostname = '127.0.0.1';
const port = 3000;
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.end('Hello World');
});
server.listen(port, hostname, () => {
console.log(`Server running at http://${hostname}:${port}/`);
});
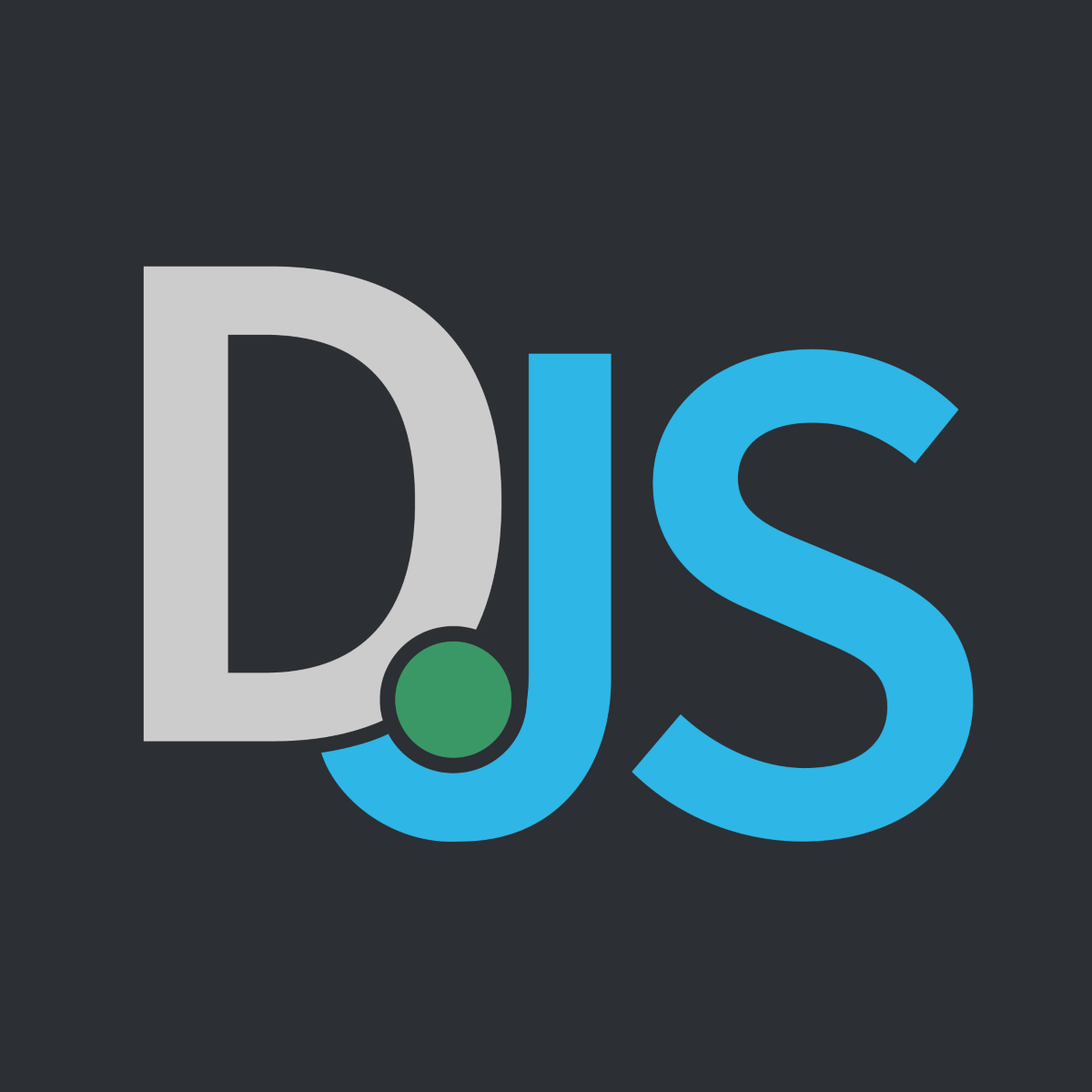
Discord.js
The bot uses discord.js to interact with the discord API.
discord.js is a powerful node.js module that allows Node to interact with the Discord API very easily. It takes a much more object-oriented approach than most other JS Discord libraries, making Node's (open source) code significantly tidier and easier to comprehend.Why?
- Object-oriented
- Speedy and efficient
- Feature-rich
- Flexible
- 100% Promise-based
const Discord = require('discord.js');
const client = new Discord.Client();
client.on('ready', () => {
console.log(`Logged in as ${client.user.tag}!`);
});
client.on('message', msg => {
if (msg.content === 'ping') {
msg.reply('Pong!');
}
});
client.login('token');
MongoDB
MongoDB is a general purpose, document-based, distributed database built for modern application developers and for the cloud era. Node uses MongoDB to store information that is used by the functions of the bot e.g. custom server prefixes and user bios.
Heroku
Heroku is a container-based cloud Platform as a Service (PaaS). Developers use Heroku to deploy, manage, and scale modern apps. Node is deployed using heroku in order to maintain a 24/7 uptime without the need to manage servers.